Excel VBA-strengesammenligning
For å sammenligne to strenger i VBA har vi en innebygd funksjon, dvs. " StrComp ". Dette kan vi lese det som “ String Comparison ”, denne funksjonen er bare tilgjengelig med VBA og er ikke tilgjengelig som et regnearkfunksjon. Den sammenligner to strenger og returnerer resultatene som “Null (0)” hvis begge strengene samsvarer, og hvis begge strengene som leveres ikke stemmer overens, vil vi få “En (1)” som resultat.
I VBA eller excel møter vi mange forskjellige scenarier. Et slikt scenario er å "sammenligne to strengverdier." I et vanlig regneark kan vi gjøre disse flere måtene, men i VBA, hvordan gjør du dette?

Nedenfor er syntaksen til “StrComp” -funksjonen.

For det første er to argumenter ganske enkle,
- for streng 1, må vi oppgi hva den første verdien vi sammenligner og
- for streng 2, må vi oppgi den andre verdien vi sammenligner.
- (Sammenlign) dette er det valgfrie argumentet til StrComp-funksjonen. Dette er nyttig når vi ønsker å sammenligne saksfølsom sammenligning. For eksempel, i dette argumentet er ikke "Excel" lik "EXCEL" fordi begge disse ordene er store og små bokstaver.
Vi kan levere tre verdier her.
- Null (0) for " Binary Compare ", dvs. "Excel", er ikke lik "EXCEL." For saksfølsom sammenligning kan vi levere 0.
- Én (1) for " Tekst sammenligne ", dvs. "Excel", er lik "EXCEL." Dette er en ikke-sakssensitiv sammenligning.
- To (2) dette bare for sammenligning av databaser.
Resultatene av “StrComp” -funksjonen er ikke SANN eller FALSK, men varierer. Nedenfor er de forskjellige resultatene av “StrComp” -funksjonen.
- Vi får "0" som resultat hvis de medfølgende strengene samsvarer.
- Vi får "1" hvis de medfølgende strengene ikke samsvarer, og i tilfelle numerisk samsvar vil vi få 1 hvis streng 1 er større enn streng 2.
- Vi får "-1" hvis streng 1-tallet er mindre enn streng 2-tallet.
Hvordan utføre streng sammenligning i VBA?
Eksempel 1
Vi vil matche " Bangalore " mot strengen " BANGALORE ."
Først erklærer du to VBA-variabler som strengen for å lagre to strengverdier.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String End Sub

Lagre to strengverdier for disse to variablene.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" End Sub
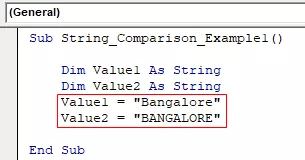
Nå erklærer du en variabel til for å lagre resultatet av “ StrComp ” -funksjonen.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String End Sub

For denne variabelen, åpne “StrComp” -funksjonen.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (End Sub
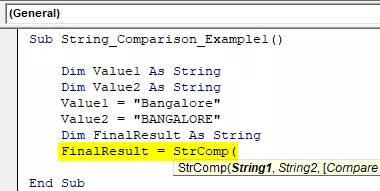
For “String1” og “String2” har vi allerede tildelt verdier gjennom variabler, så skriv inn henholdsvis variabelnavn.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, End Sub
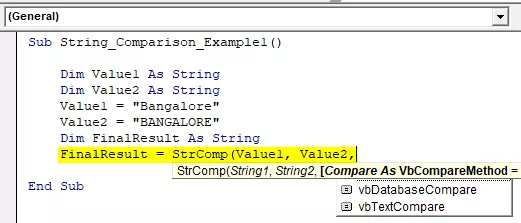
Den siste delen av funksjonen er "Sammenlign" for dette valget "vbTextCompare."
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) End Sub

Now show the “Final Result” variable in the message box in VBA.
Code:
Sub String_Comparison_Example1() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub

Ok, let’s run the code and see the result.
Output:

Since both the strings “Bangalore” and “BANGALORE” are the same, we got the result as 0, i.e., matching. Both the values are case sensitive since we have supplied the argument as “vbTextCompare” it has ignored case sensitive match and matched only values, so both the values are the same, and the result is 0, i.e., TRUE.
Code:
Sub String_Comparison_Example1() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub

Example #2
For the same code, we will change the compare method from “vbTextCompare” to “vbBinaryCompare.”
Code:
Sub String_Comparison_Example2() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now run the code and see the result.
Output:

Even though both the strings are the same, we got the result as 1, i.e., Not Matching because we have applied the compare method as “vbBinaryCompare,” which compares two values as case sensitive.
Example #3
Now we will see how to compare numerical values. For the same code, we will assign different values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Both the values are 500, and we will get 0 as a result because both the values are matched.
Output:
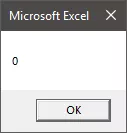
Now I will change the Value1 number from 500 to 100.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:
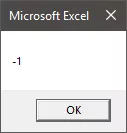
We know Value1 & Value2 aren’t the same, but the result is -1 instead of 1 because for numerical comparison when the String 1 value is greater than String 2, we will get this -1.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now I will reverse the values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 1000 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:

This is not special. If not match, we will get 1 only.
Things to Remember here
- (Compare) argument of “StrComp” is optional, but in case of case sensitive match, we can utilize this, and the option is “vbBinaryCompare.”
- The result of numerical values is slightly different in case String 1 is greater than string 2, and the result will be -1.
- Results are 0 if matched and 1 if not matched.
Recommended Articles
Dette har vært en guide til VBA-strengesammenligning. Her diskuterer vi hvordan man kan sammenligne to strengverdier ved hjelp av StrComp-funksjonen i excel VBA sammen med eksempler og laste ned en excel-mal. Du kan også ta en titt på andre artikler relatert til Excel VBA -
- Veiledning til VBA-strengfunksjoner
- VBA Split String in Array
- VBA SubString Methods
- VBA Tekst